Overview
- In this blog, you will explore what array means in C programming
- We will look at declaring arrays, initializing arrays, using arrays in 1-D and 2-D, and passing arrays to functions.
- By this blog, we will simply build your knowledge about C programming starting from scratch
An array is a variable that can store multiple values. For example, if you want to store 100 integers, you can create an array for it.
int data[10];
How to declare an array?
- Array variables are declared identically to variables of their data type, except that the variable name is followed by one pair of square [ ] brackets for each dimension of the array.
- Uninitialized arrays must have the dimensions of their rows, columns, etc. listed within the square brackets.
- Dimensions used when declaring arrays in C must be positive integral constants or constant expressions.
- In C99, dimensions must still be positive integers, but variables can be used, so long as the variable has a positive value at the time the array is declared. ( Space is allocated only once, at the time the array is declared. The array does NOT change sizes later if the variable used to declare it changes. )
int i, j, intArray[ 10 ], number; float floatArray[ 1000 ]; int tableArray[ 3 ][ 5 ]; /* 3 rows by 5 columns */ const int NROWS = 100; // ( Old code would use #define NROWS 100 ) const int NCOLS = 200; // ( Old code would use #define NCOLS 200 ) float matrix[ NROWS ][ NCOLS ];
Another example comes with using mark, an array of floating-point type.
float mark[5];
Its size is 5. Meaning, it can hold 5 floating-point values.
Access Array Elements
You can access elements of an array by indices.An element is accessed by indexing the array name. This is done by placing the index of the element within square brackets after the name of the array.
For example, you declared an array mark as above. The first element is mark[0]
, the second element is mark[1]
and so on.
Initializing Arrays
Arrays may be initialized when they are declared, just as any other variables. Place the initialization data in curly {} braces following the equals sign. Note the use of commas in the examples below. An array may be partially initialized, by providing fewer data items than the size of the array. The remaining array elements will be automatically initialized to zero. If an array is to be completely initialized, the dimension of the array is not required. The compiler will automatically size the array to fit the initialized data.
int i = 5, intArray[ 6 ] = { 1, 2, 3, 4, 5, 6 }, k; float sum = 0.0f, floatArray[ 100 ] = { 1.0f, 5.0f, 20.0f }; double piFractions[ ] = { 3.141592654, 1.570796327, 0.785398163 };
You can also initialize an array like this.
int mark[] = {19, 10, 8, 17, 9};
Here, we haven’t specified the size. However, the compiler knows its size is 5 as we are initializing it with 5 elements.
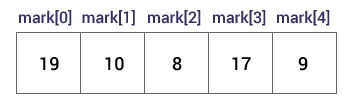
mark[0] is equal to 19 mark[1] is equal to 10 mark[2] is equal to 8 mark[3] is equal to 17 mark[4] is equal to 9
Using Arrays
- Elements of an array are accessed by specifying the index ( offset ) of the desired element within square [ ] brackets after the array name.
- Array subscripts must be of integer type. ( int, long int, char, etc. )
- VERY IMPORTANT: Array indices start at zero in C, and go to one less than the size of the array. For example, a five element array will have indices zero through four. This is because the index in C is actually an offset from the beginning of the array. ( The first element is at the beginning of the array, and hence has zero offset. )
- Landmine: The most common mistake when working with arrays in C is forgetting that indices start at zero and stop one less than the array size.
- Arrays are commonly used in conjunction with loops, in order to perform the same calculations on all ( or some part ) of the data items in the array.
1-D Arrays
/* Program to calculate the first 20 Fibonacci numbers. */ #include <stdlib.h> #include <stdio.h> int main( void ) { int i, fibonacci[ 20 ]; fibonacci[ 0 ] = 0; fibonacci[ 1 ] = 1; for( i = 2; i < 20; i++ ) fibonacci[ i ] = fibonacci[ i - 2 ] + fibonacci[ i - 1 ]; for( i = 0; i < 20; i++ ) printf( "Fibonacci[ %d ] = %f\n", i, fibonacci[ i ] ); } /* End of sample program to calculate Fibonacci numbers */
Here’s how you can get input/output of the arrays.
// Program to take 5 values from the user and store them in an array // Print the elements stored in the array #include <stdio.h> int main() { int values[5]; printf("Enter 5 integers: "); // taking input and storing it in an array for(int i = 0; i < 5; ++i) { scanf("%d", &values[i]); } printf("Displaying integers: "); // printing elements of an array for(int i = 0; i < 5; ++i) { printf("%d\n", values[i]); } return 0; }
Access elements out of its bound!
Suppose you declared an array of 10 elements. Let’s say,
int testArray[10];
You can access the array elements from testArray[0]
to testArray[9]
.
Now let’s say if you try to access testArray[12]
. The element is not available. This may cause unexpected output (undefined behavior). Sometimes you might get an error and some other time your program may run correctly.
Hence, you should never access elements of an array outside of its bound.
2-D Arrays
/* Sample program Using 2-D Arrays */ #include <stdlib.h> #include <stdio.h> int main( void ) { /* Program to add two multidimensional arrays */ /* Written May 1995 by George P. Burdell */ int a[ 2 ][ 3 ] = { { 5, 6, 7 }, { 10, 20, 30 } }; int b[ 2 ][ 3 ] = { { 1, 2, 3 }, { 3, 2, 1 } }; int sum[ 2 ][ 3 ], row, column; /* First the addition */ for( row = 0; row < 2; row++ ) for( column = 0; column < 3; column++ ) sum[ row ][ column ] = a[ row ][ column ] + b[ row ][ column ]; /* Then print the results */ printf( "The sum is: \n\n" ); for( row = 0; row < 2; row++ ) { for( column = 0; column < 3; column++ ) printf( "\t%d", sum[ row ][ column ] ); printf( '\n' ); /* at end of each row */ } return 0; }
Passing Arrays as Function Arguments in C
If you want to pass a single-dimension array as an argument in a function, you would have to declare a formal parameter in one of following three ways and all three declaration methods produce similar results because each tells the compiler that an integer pointer is going to be received. Similarly, you can pass multi-dimensional arrays as formal parameters. Here’s the example of formal parameters as a pointer.
void myFunction(int *param) { . . . }
Now, consider the following function, which takes an array as an argument along with another argument and based on the passed arguments, it returns the average of the numbers passed through the array as follows.
double getAverage(int arr[], int size) { int i; double avg; double sum = 0; for (i = 0; i < size; ++i) { sum += arr[i]; } avg = sum / size; return avg; }
Now, let us call the above function as follows.
#include <stdio.h> /* function declaration */ double getAverage(int arr[], int size); int main () { /* an int array with 5 elements */ int balance[5] = {1000, 2, 3, 17, 50}; double avg; /* pass pointer to the array as an argument */ avg = getAverage( balance, 5 ) ; /* output the returned value */ printf( "Average value is: %f ", avg ); return 0; }
When the above code is compiled together and executed, it produces the following result.
Average value is: 214.400000